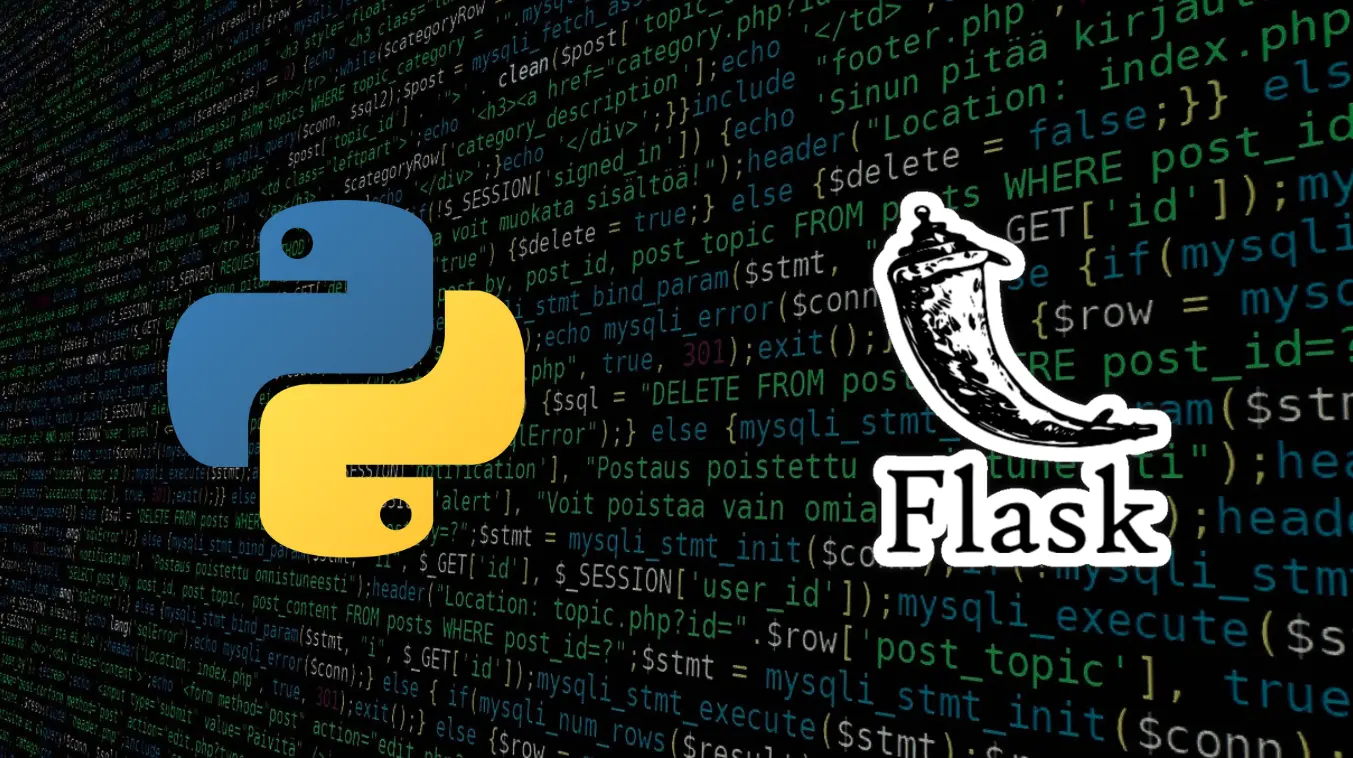
Python Flask: Basic App
How to build a Basic Python Flask Application
Python Flask is a lightweight web framework that gives you the flexibility to start small and grow fast. In this tutorial, we’ll walk through installing Flask, setting up routes, handling HTTP methods, creating middleware, and implementing simple server-side rendering (SSR).
- Installation Before you begin, ensure you have Python installed (preferably Python 3.6+). You can install Flask using pip. Open your terminal and run:
pip install Flask
This command installs Flask and its dependencies. It’s a minimalistic approach that allows you to start coding right away.
Flask’s simplicity makes it an excellent choice for beginners and advanced developers alike.
- Setting Up Your Flask Application Create a new file called app.py. This file will be the main entry point for your application. Here’s a simple starting point:
from flask import Flask, request, render_template, jsonify
app = Flask(__name__)
@app.route("/")
def home():
return "<h1>Welcome to your Flask App!</h1>"
if __name__ == "__main__":
app.run(debug=True)
This simple script creates an instance of the Flask application and defines a single route (/). By calling app.run(debug=True), you enable debug mode which helps during development.
- Creating Multiple Routes Let’s add a couple of routes to your application. One route will handle a GET request, and another will support multiple HTTP methods (GET and POST).
Static Route Add a route for an about page:
@app.route("/about")
def about():
return "<h2>This is the About page of our Flask App!</h2>"
Dynamic Route with HTTP Methods Now create a route that handles both GET and POST requests. This is useful when you want the same URL endpoint to handle displaying a form and processing its data.
@app.route("/submit", methods=["GET", "POST"])
def submit():
if request.method == "POST":
# Process data from a form submission
name = request.form.get("name", "Guest")
return f"<h3>Thanks for your submission, {name}!</h3>"
return '''
<form method="POST">
Name: <input type="text" name="name">
<input type="submit" value="Submit">
</form>
'''
In this example, if the client sends a GET request, the server displays an HTML form. On submission (a POST request), it processes the data and responds accordingly.
Using a single endpoint to handle multiple HTTP methods is common in RESTful designs.
- Adding Middleware Middleware in Flask allows you to execute logic before or after a request is processed. Flask provides the before_request and after_request decorators for this purpose.
Before Request Middleware This function runs before every request, which is perfect for tasks like initializing variables or checking user authentication.
@app.before_request
def before_request_func():
print("A request is about to be handled.")
After Request Middleware This function is executed after the request is processed. It’s useful for cleaning up resources or modifying the response.
@app.after_request
def after_request_func(response):
response.headers["X-Custom-Header"] = "FlaskMiddleware"
return response
Middleware is a powerful concept that lets you inject extra logic seamlessly into the request/response cycle.
- Server-Side Rendering (SSR) For server-side rendering, Flask uses its render_template function, which integrates well with the Jinja2 templating engine. This approach enables you to separate HTML (presentation) from your Python logic.
Setting Up Templates Create a directory called templates in the same location as your app.py.
Inside templates, create a file named index.html:
<!-- templates/index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Flask SSR Example</title>
</head>
<body>
<h1>{{ greeting }}</h1>
<p>Welcome to the server rendered page of the Flask app.</p>
</body>
</html>
Update your route to render this template:
@app.route("/ssr")
def server_side_rendering():
data = {"greeting": "Hello, SSR World!"}
return render_template("index.html", **data)
Server-side rendering is advantageous for better SEO and faster initial page load times, as the HTML is ready to be served immediately.
- Running Your App Finally, run your application:
bash Copy Edit python app.py Visit the following URLs to see your routes in action:
http://127.0.0.1:5000/ – Home page.
http://127.0.0.1:5000/about – About page.
http://127.0.0.1:5000/submit – Form submission page.
http://127.0.0.1:5000/ssr – Server-side rendered page.
Final Thoughts
This quick tutorial covers the essentials of setting up a Flask application, defining multiple routes, handling various HTTP methods, integrating middleware, and implementing SSR through template rendering. This foundation allows you to build more complex applications by exploring additional Flask features and extensions.
Experiment with adding more routes, improving middleware logic, or incorporating a database for dynamic data handling. Flask’s simplicity makes it a great tool for both beginners and experienced developers.
Happy coding with Flask!
More Articles
Key Words:
Flaskpythonweb serverserverbackend