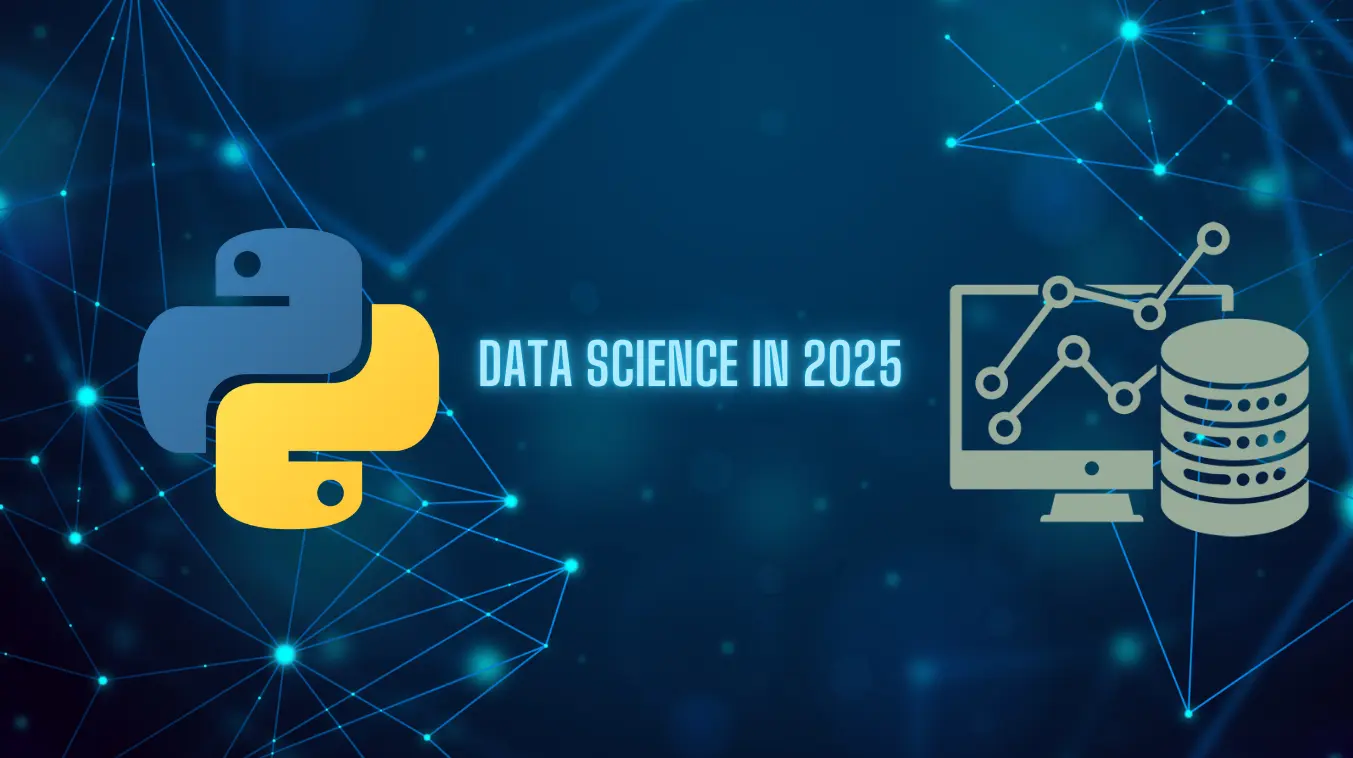
Master Data Science In 2025
Mastering Data Science and Visualization with Python: A Roadmap
Data science is an ever-evolving domain that blends statistics, programming, and domain expertise to extract insights from data. Whether you’re an aspiring data scientist or a professional looking to polish your skills, understanding the full cycle—from data collection, cleaning, and preprocessing to visualization and modeling—is crucial. In this blog post, we’ll walk you through key concepts, definitions, and practical code examples that will help you become proficient in data science and visualization using Python.
What Is Data Science?
Data science is an interdisciplinary field that focuses on extracting meaningful insights from large, often unstructured, sets of data. It involves various processes including:
-
Data Cleaning: The practice of removing corrupt or inaccurate records.
-
Data Preprocessing: Transforming raw data into a format that is more appropriate for analysis.
-
Exploratory Data Analysis (EDA): Summarizing a dataset’s main characteristics, often using visual methods.
-
Modeling: Building predictive or descriptive models.
-
Visualization: Presenting data in an interpretable and aesthetic form.
The power of data science lies in its ability to convert data into actionable insights, driving better decision-making across various industries.
Laying the Foundation: Your Essential Python Libraries
Before diving into data manipulation and visualization, ensure you have the following libraries installed. You can install them using pip:
pip install numpy pandas matplotlib seaborn scikit-learn
-
NumPy: Provides support for arrays and high-performance numerical operations.
-
Pandas: Offers data structures like DataFrames, ideal for handling tabular data.
-
Matplotlib: A versatile plotting library that helps create a wide range of static, animated, and interactive visualizations.
-
Seaborn: Built on top of Matplotlib, it offers a higher-level interface for creating attractive statistical graphics.
-
Scikit-learn: A powerful library for machine learning, providing simple and efficient tools for data mining and data analysis.
Basic Data Cleaning and Preprocessing
Data cleaning and preprocessing are often the most time-consuming steps in any data science project, but they are fundamental to ensuring your analysis is accurate and your models are effective.
Understanding Common Data Issues
-
Missing Values: Incomplete data entries can skew analysis if not handled properly.
-
Inconsistent Data Types: Mismatched data types (e.g., numbers stored as strings) can complicate analysis.
-
Outliers: Extreme values may result from data entry errors or natural variability.
-
Duplicated Data: Duplicate records can introduce bias in your analysis.
A Step-by-Step Guide with Code Examples Here’s how you can tackle these common problems using Python and Pandas:
- Loading Your Data
import pandas as pd
# Load a sample dataset
df = pd.read_csv('sample_dataset.csv')
print(df.head())
- Handling Missing Values
# Check for missing values
print(df.isnull().sum())
# Example strategy: Fill missing values with the mean (for numerical columns)
df['numerical_column'].fillna(df['numerical_column'].mean(), inplace=True)
# For categorical data: You might fill missing values with the mode
df['categorical_column'].fillna(df['categorical_column'].mode()[0], inplace=True)
- Data Type Conversion
# Convert column types if necessary
df['date_column'] = pd.to_datetime(df['date_column'])
- Removing Duplicates
# Remove duplicate rows
df.drop_duplicates(inplace=True)
- Outlier Detection (using box plots)
import seaborn as sns
import matplotlib.pyplot as plt
# Visualize outliers in a numerical column
sns.boxplot(x=df['numerical_column'])
plt.title('Box Plot for Numerical Column')
plt.show()
Advanced Data Cleaning and Preprocessing
Beyond the basics of handling missing values, converting data types, and basic outlier visualization, several advanced techniques can refine your dataset to a state that’s robust for analysis or visualization. This section details additional strategies for further cleaning and preparing your data.
1. Scaling and Normalizing Data
Scaling and normalization ensure that numerical features share a common scale, which is especially useful when you compare features with varying ranges. While these techniques are often associated with machine learning preprocessing, they are equally valuable for statistical analysis and visualization.
Standardization and Min-Max Scaling You can standardize your data (transforming features to have zero mean and unit variance) or apply Min-Max scaling (scaling data to a fixed range, often 0–1). Even without applying machine learning, these adjustments allow clearer data comparisons.
import pandas as pd
from sklearn.preprocessing import StandardScaler, MinMaxScaler
# Sample DataFrame with numerical columns
df = pd.DataFrame({
'feature1': [10, 20, 30, 40, 50],
'feature2': [100, 150, 200, 250, 300]
})
# Standardization: zero mean, unit variance
scaler_std = StandardScaler()
df[['feature1_std', 'feature2_std']] = scaler_std.fit_transform(df[['feature1', 'feature2']])
# Min-Max Scaling: transform values between 0 and 1
scaler_minmax = MinMaxScaler()
df[['feature1_minmax', 'feature2_minmax']] = scaler_minmax.fit_transform(df[['feature1', 'feature2']])
print(df)
Log Transformation For data with a skewed distribution, applying a log transformation can make patterns more apparent.
import numpy as np
# Log transform a positively skewed column
df['feature1_log'] = np.log1p(df['feature1']) # log1p handles zero values gracefully
print(df[['feature1', 'feature1_log']])
2. Advanced Data Cleaning Techniques
a. Removing and Imputing Outliers While visualization techniques can flag outliers, you can also programmatically detect them. The IQR (Interquartile Range) method is a common approach.
# Define a function to remove outliers based on the IQR method
def remove_outliers(dataframe, column):
Q1 = dataframe[column].quantile(0.25)
Q3 = dataframe[column].quantile(0.75)
IQR = Q3 - Q1
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
return dataframe[(dataframe[column] >= lower_bound) & (dataframe[column] <= upper_bound)]
# Apply the function to remove outliers from a numerical column
df_clean = remove_outliers(df, 'feature1')
print(df_clean)
Alternatively, instead of removing outliers, consider imputing them with a measure of central tendency (mean or median) if outlier handling is critical for your analysis.
b. Handling Categorical Data For textual or categorical features, cleaning might include standardizing case, stripping unwanted spaces, or consolidating similar categories.
# Example: Standardize categorical entries by trimming whitespace and converting to lowercase
df['category'] = ['Apple ', ' banana', 'APPLE', 'Banana ', 'Cherry']
df['category_clean'] = df['category'].str.strip().str.lower()
print(df[['category', 'category_clean']])
c. Cleaning Text Data with Regular Expressions When dealing with free-form text data, regex (regular expressions) can help remove unwanted characters or patterns.
import re
# Example function to clean text by removing non-alphanumeric characters
def clean_text(text):
return re.sub(r'[^A-Za-z0-9 ]+', '', text)
df['text'] = ['Hello, World!', 'Pandas is #1.', 'Data-driven decisions!!!']
df['text_clean'] = df['text'].apply(clean_text)
print(df[['text', 'text_clean']])
d. Imputing Values Using Interpolation For time-series or sequential data, Pandas provides powerful interpolation methods to fill in missing values.
# Sample DataFrame with missing values in a time series
df_time = pd.DataFrame({
'date': pd.date_range(start='2025-01-01', periods=6, freq='D'),
'value': [10, np.nan, 15, np.nan, 20, 25]
})
df_time.set_index('date', inplace=True)
# Interpolate missing values (linear interpolation)
df_time['value_interp'] = df_time['value'].interpolate(method='linear')
print(df_time)
3. Leveraging Advanced Pandas Operations
Advanced Pandas functionalities not only make cleaning more robust but also enable efficient data transformation and reshaping.
a. Grouping and Aggregation Use groupby to aggregate data by categories or time frames for deeper insights.
# Grouping data and calculating summary statistics
group_summary = df.groupby('category_clean')['feature1'].agg(['mean', 'min', 'max'])
print(group_summary)
b. Pivot Tables Pivot tables allow you to reorganize data for easier analysis or reporting.
# Creating a pivot table
data = {
'date': pd.date_range(start='2025-01-01', periods=8, freq='D'),
'category': ['A', 'A', 'B', 'B', 'A', 'B', 'A', 'B'],
'value': [5, 3, 6, 7, 2, 4, 8, 5]
}
df_pivot = pd.DataFrame(data)
pivot_table = df_pivot.pivot_table(values='value', index='date', columns='category', aggfunc='sum')
print(pivot_table)
c. Merging and Joining Datasets Combining datasets is often needed to enhance your analysis. Pandas provides methods such as merge, join, and concat.
# Merge two sample DataFrames using a common key
df1 = pd.DataFrame({'id': [1, 2, 3], 'value1': ['A', 'B', 'C']})
df2 = pd.DataFrame({'id': [1, 2, 4], 'value2': ['D', 'E', 'F']})
df_merged = pd.merge(df1, df2, on='id', how='outer')
print(df_merged)
d. Working with Multi-Index DataFrames Handling complex datasets with multiple dimensions can be managed effectively using MultiIndex objects.
# Create a multi-index DataFrame
arrays = [['A', 'A', 'B', 'B'], ['one', 'two', 'one', 'two']]
index = pd.MultiIndex.from_arrays(arrays, names=('letter', 'number'))
df_multi = pd.DataFrame({'values': [10, 20, 30, 40]}, index=index)
print(df_multi)
# Accessing data by level
print(df_multi.xs('A', level='letter'))
Exploring Data Visualization
Visualization is not only about making data look appealing; it’s about revealing patterns, trends, and outliers which might be buried within raw data. With Python, visualization becomes approachable with several libraries designed to make your insights pop.
Basic Visualization Techniques Histogram
# Importing matplotlib for histogram
import matplotlib.pyplot as plt
plt.hist(df['numerical_column'], bins=30, color='skyblue', edgecolor='black')
plt.title('Histogram of Numerical Column')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.show()
Scatter Plot
plt.scatter(df['feature_1'], df['feature_2'], alpha=0.5)
plt.title('Scatter Plot Between Feature 1 and Feature 2')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.show()
Correlation Heatmap
correlation_matrix = df.corr()
sns.heatmap(correlation_matrix, annot=True, cmap='coolwarm')
plt.title('Correlation Heatmap')
plt.show()
These visualizations give you insight into the distribution of your data as well as the relationships between different features. Tweaking parameters like colors, labels, and transparency can further enhance your plots and make them publication-ready.
Bringing It All Together: A Roadmap
Step 1: Learn the Fundamentals
-
Mathematics & Statistics: Start with basic statistics and probability. Understanding concepts like mean, median, standard deviation, and hypothesis testing is crucial.
-
Programming in Python: Familiarize yourself with Python, and learn how to manipulate data using libraries like NumPy and Pandas.
Step 2: Data Wrangling and Preprocessing
-
Handling Missing Data: Learn techniques to fill or drop missing values.
-
Data Transformation: Practice converting data types and creating new features.
-
Outlier Detection: Understand how to identify and deal with outliers.
Step 3: Exploratory Data Analysis (EDA)
-
Descriptive Statistics: Use Pandas to compute summary statistics.
-
Visualization: Utilize Matplotlib and Seaborn to plot data, uncover patterns, and spot anomalies.
-
Feature Engineering: Experiment with creating new features that can improve model performance.
Step 4: Modeling and Evaluation
-
Machine Learning: Start with simple models in scikit-learn, such as linear regression and decision trees.
-
Validation Techniques: Learn about cross-validation and metrics like accuracy, precision, and recall.
-
Iterative Improvement: Fine-tune your models and experiment with advanced algorithms.
Step 5: Advanced Visualization Techniques
-
Interactive Visualizations: Libraries like Plotly and Bokeh allow you to create dynamic visualizations for more engaging presentations.
-
Storytelling with Data: Develop the skill to narrate a story with your data; always connect the visuals with a compelling narrative.
Step 6: Build a Portfolio
-
Projects: Work on real datasets, whether from open data sources or through Kaggle competitions.
-
Blogs and Tutorials: Sharing your work publicly through blogs or tutorials not only reinforces your learning but also attracts feedback from the community.
-
GitHub Repository: Document your projects on GitHub to create a professional portfolio that showcases your skills and expertise.
Code Tips for a Successful Data Science Journey
-
Modularity: Write modular code; break your process into functions and classes that can be reused.
-
Documentation: Comment your code and maintain clear, readable notebooks or scripts.
-
Version Control: Use Git for version control to manage changes in your projects.
-
Community Engagement: Participate in data science forums and communities to learn from your peers and stay updated on best practices.
Final Thoughts
Embarking on a data science journey with Python is both exciting and challenging. By following this roadmap—starting with strong fundamentals, diving into data cleaning and preprocessing, exploring visualization techniques, and finally building projects—you can systematically master the craft. With consistent practice and a willingness to learn, the field of data science offers limitless possibilities to transform data into insights that drive decision-making.
Business Insider ISHIR Ikangai ZDNETMore Articles
Key Words:
Data Sciencepythonpandasdata preprocessingvisualization