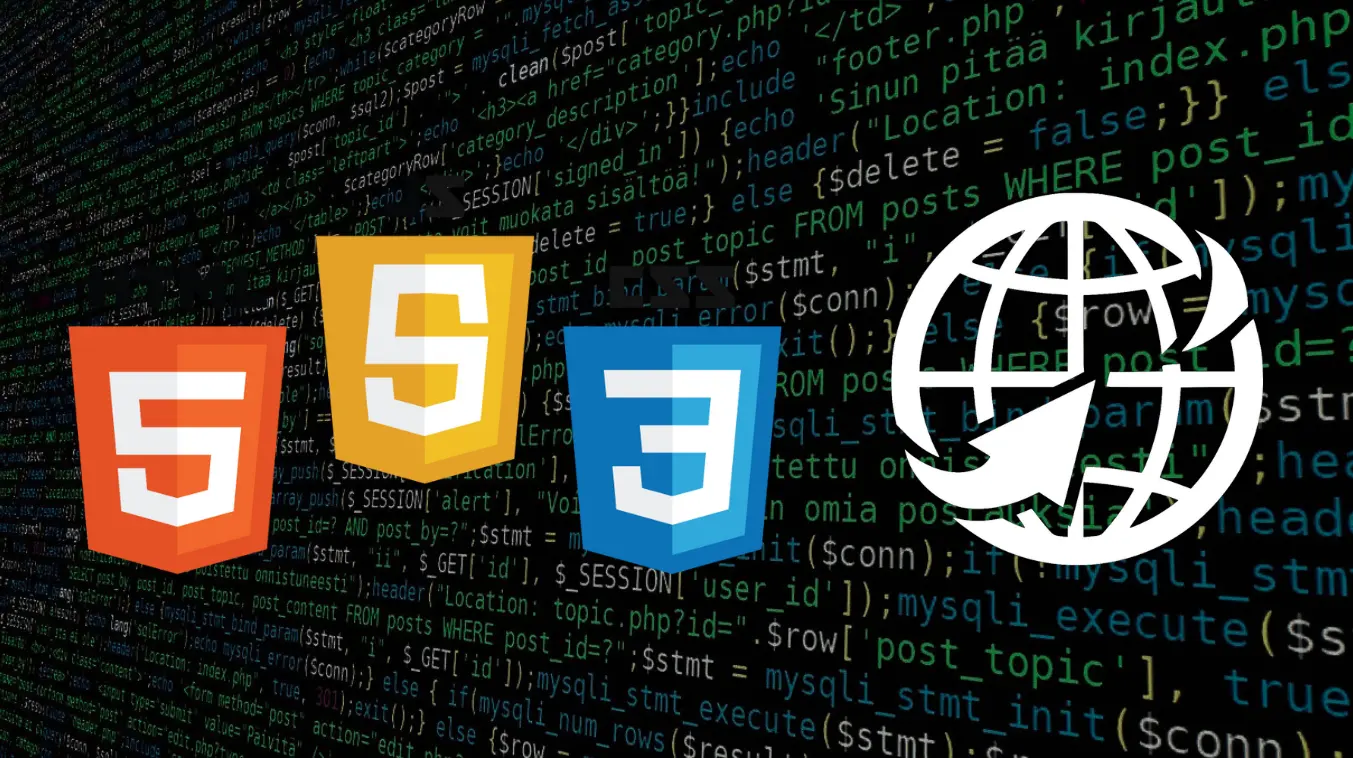
Javascript DOM Manipulation
Mastering Advanced DOM Manipulation & JavaScript Events
In modern web development, controlling the Document Object Model (DOM) with JavaScript is crucial for building responsive and interactive applications. While basic manipulation (like changing text or adding an element) is often covered in introductory resources, mastering advanced techniques can significantly improve your coding effectiveness—especially when preparing for technical interviews or scaling up dynamic applications.
In this tutorial, we will:
-
Recap what the DOM is
-
Review fundamental methods for DOM manipulation
-
Explore advanced event handling techniques such as event delegation, propagation control, and animations
-
Build a dynamic UI component with vanilla JavaScript
Let’s jump in!
Understanding the DOM
The DOM is a tree-like representation of your HTML document where each element (tags, text, attributes) becomes a node. JavaScript uses the DOM to access, modify, and animate content on the fly. The methods querySelector() and querySelectorAll() (among others) let you select elements with familiar CSS selectors, making it easier to integrate powerful dynamic behavior with your HTML structure. developer.mozilla.org
Basic DOM Manipulation Recap
Before we embrace the advanced topics, it’s important to have a quick refresher on selecting and modifying elements. For example, consider the snippet:
<!-- HTML -->
<div id="content">
<p class="info">Welcome to the site!</p>
</div>
// JavaScript
const infoParagraph = document.querySelector('.info');
infoParagraph.textContent = 'Hello, dynamic world!';
This simple change updates the paragraph text. But as the interface grows, basic methods quickly become insufficient. That’s where advanced techniques come in.
Advanced DOM Manipulation Techniques
- Dynamic Element Creation & Cloning Creating elements on the fly is a common practice. Use document.createElement() to build new nodes and inject them into the DOM. Cloning elements with cloneNode(true) can be especially useful when you need to duplicate complex components.
// Create a new card element
const card = document.createElement('div');
card.className = 'card';
card.innerHTML = `
<h2>New Card Title</h2>
<p>This card was created dynamically!</p>
`;
document.body.appendChild(card);
// Clone an element (deep clone)
const clonedCard = card.cloneNode(true);
clonedCard.querySelector('h2').textContent = 'Cloned Card';
document.body.appendChild(clonedCard);
This approach keeps your code modular and allows you to build dynamic interfaces on the fly.
- Event Handling: Advanced Patterns & Delegation When your UI has many interactive elements, attaching event listeners to each element individually can lead to performance bottlenecks and redundant code. Event Delegation solves this by leveraging event bubbling: you attach a listener to a common parent element and handle events that “bubble up” from child elements.
// Parent container that holds many items
const listContainer = document.getElementById('list-container');
// Event delegation: handle click for any element with the 'item' class
listContainer.addEventListener('click', (event) => {
if (event.target && event.target.matches('.item')) {
console.log('Item clicked:', event.target.textContent);
// Optionally, prevent further propagation if needed:
// event.stopPropagation();
}
});
This strategy not only reduces the number of event listeners but also neatly handles dynamically added elements.
Managing Event Propagation
To refine user interactions, you may need to prevent the default behavior or stop an event from propagating (bubbling up). Use:
-
event.preventDefault() to cancel the default action.
-
event.stopPropagation() to stop the event from reaching parent elements.
-
event.stopImmediatePropagation() to halt other event handlers on the same element.
const button = document.getElementById('action-btn');
button.addEventListener('click', (event) => {
event.preventDefault();
event.stopPropagation();
alert('Button clicked, propagation stopped!');
});
Understanding these methods is key when designing complex UIs where multiple interactions might conflict.
- Animating the DOM Animations can breathe life into your web app. You have two common avenues:
a. CSS Transitions with JavaScript Triggering You can define CSS animations and then trigger them by adding or removing classes.
<!-- CSS -->
<style>
.fade {
opacity: 0;
transition: opacity 1s ease-in-out;
}
.fade.visible {
opacity: 1;
}
</style>
<div id="animBox" class="fade">Watch me fade in!</div>
// JavaScript to add the class on a delay
const animBox = document.getElementById('animBox');
setTimeout(() => {
animBox.classList.add('visible');
}, 1000);
This method leverages the browser’s optimized CSS engine for smooth transitions.
b. Animations Using requestAnimationFrame For more granular control over animations (such as smooth movement), requestAnimationFrame is ideal.
function slideElement(element, distance) {
let pos = 0;
function animate() {
pos += 2; // Adjust speed by modifying the increment
element.style.transform = `translateX(${pos}px)`;
if (pos < distance) {
requestAnimationFrame(animate);
}
}
requestAnimationFrame(animate);
}
const movingBox = document.getElementById('moving-box');
slideElement(movingBox, 200);
Using requestAnimationFrame synchronizes your animation with the browser’s refresh rate, resulting in smoother visuals.
- Building Dynamic UI Components As your application grows, you might need to construct custom components—like an accordion, modal dialog, or tabbed interface—from scratch.
Example: Accordion Component
<!-- HTML Structure -->
<div class="accordion">
<div class="accordion-header">Section 1</div>
<div class="accordion-panel">Content for section 1</div>
<div class="accordion-header">Section 2</div>
<div class="accordion-panel">Content for section 2</div>
</div>
// JavaScript Component
class Accordion {
constructor(container) {
this.container = container;
this.headers = container.querySelectorAll('.accordion-header');
this.headers.forEach(header => {
header.addEventListener('click', () => this.togglePanel(header));
});
}
togglePanel(header) {
const panel = header.nextElementSibling;
// Toggle the panel visibility with a class change
panel.classList.toggle('active');
}
}
// Initialize the accordion
const accordionContainer = document.querySelector('.accordion');
const accordion = new Accordion(accordionContainer);
And a bit of CSS to smooth it out:
.accordion-panel {
display: none;
transition: max-height 0.3s ease;
}
.accordion-panel.active {
display: block;
}
This example demonstrates how to encapsulate behavior within a JavaScript class, paving the way to more modular and maintainable code.
-
Additional Advanced Tips Virtual DOM Concepts: While libraries like React abstract the DOM behind a “virtual” version, understanding real DOM manipulations can help optimize your performance—even when using modern frameworks.
-
Performance Considerations: Batch your DOM updates when possible (for example, use documentFragment for creating multiple elements off-screen), and keep in mind that excessive event listeners or frequent DOM reflows can be costly.
-
Debugging Tools: Use browser developer tools to inspect the DOM tree and monitor event propagation. Tools like the “Elements” inspector and “Event Listeners” pane are invaluable when debugging complex interactions.
Conclusion
By mastering advanced DOM manipulation and sophisticated event handling, you gain finer control over your web interfaces and improve user experiences significantly. Whether you’re building dynamic animations, complex UI components, or simply streamlining event management through delegation, these techniques will help elevate your skill set to the next level. Practice these strategies by building small projects—like dynamic shopping lists or interactive accordions—to consolidate your learning.
Happy coding!
More Articles
Key Words:
JavascriptDOMfront end developerecmascript