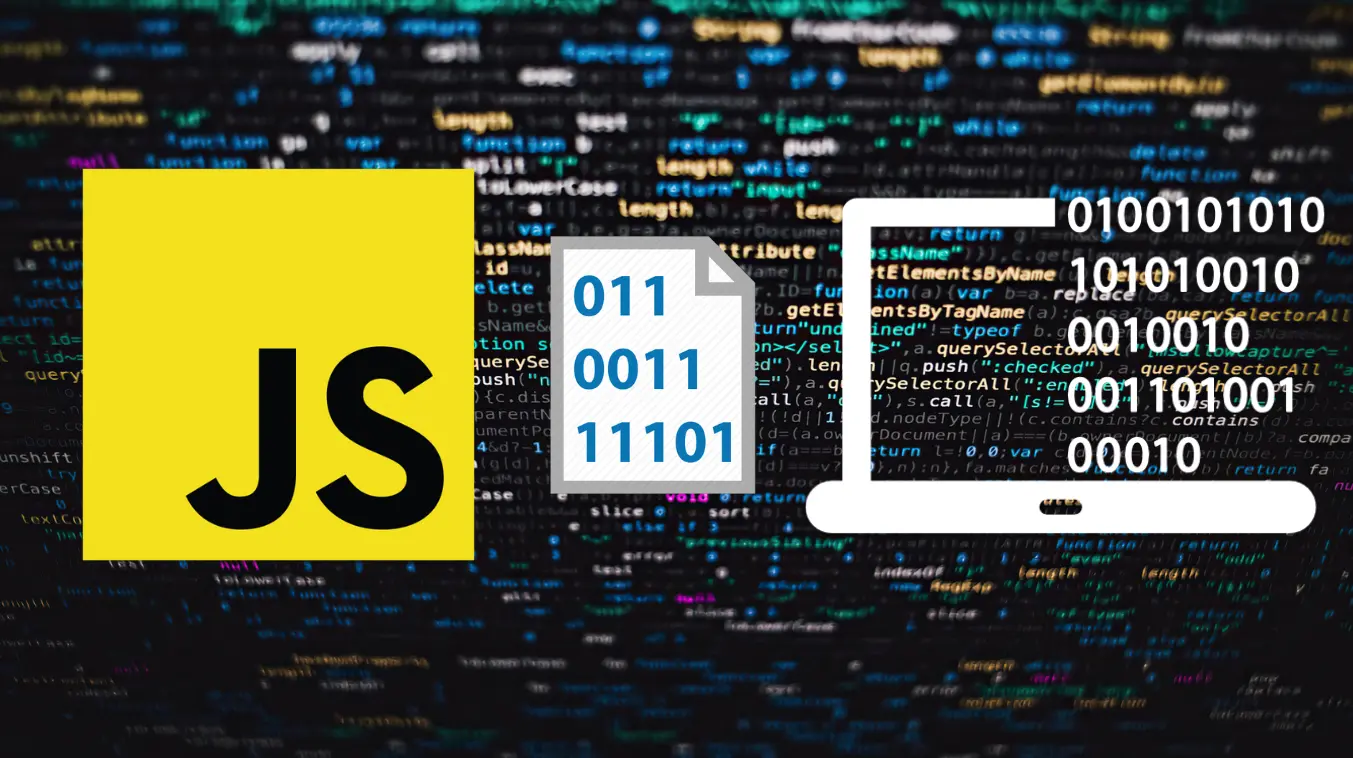
Javascript With Buffers & Binary
Javascirpt With Buffers & Binary
Understanding Binary and Buffers
JavaScript represents numbers internally as 64‑bit floating point, but provides ArrayBuffer to work with raw bytes directly . An ArrayBuffer is a contiguous chunk of memory, essentially a “byte array,” which you can’t access directly but only through views like TypedArrays or DataView . TypedArrays (e.g., Uint8Array, Int32Array) provide array‑like access to the buffer’s bytes, each entry representing one element of a specific bit‑width and signedness** .**
// Create a 16‑byte buffer and view it as bytes
const buffer = new ArrayBuffer(16);
const bytes = new Uint8Array(buffer);
console.log(bytes.length); // 16
Working with ArrayBuffers and TypedArrays
To read or write multi‑byte values (e.g., 32‑bit integers or 64‑bit floats) with explicit endianness control, use DataView __developer.mozilla.org . This is especially useful when handling binary formats or communicating with systems that use a specific byte order (big‑endian vs. little‑endian) __developer.mozilla.org .
// Write and read a 32‑bit unsigned integer at byte offset 0
const dv = new DataView(buffer);
dv.setUint32(0, 0xdeadbeef, true); // little-endian
console.log(dv.getUint32(0, true)); // 3735928559
Text Encoding and Decoding with TextEncoder/TextDecoder
Converting between JavaScript strings (UTF‑16) and UTF‑8 byte sequences is straightforward with TextEncoder and TextDecoder .
// Encode a string to UTF‑8 bytes
const encoder = new TextEncoder();
const utf8bytes = encoder.encode("Hello, 👋");
// Decode UTF‑8 bytes back to string
const decoder = new TextDecoder();
const decoded = decoder.decode(utf8bytes);
console.log(decoded); // "Hello, 👋"
-
TextEncoder’s .encode() returns a Uint8Array of UTF‑8 bytes .
-
TextDecoder’s .decode() converts an ArrayBuffer or view back to a string .
Base64 Encoding and Decoding with btoa/atob
For text‑based systems or URLs that only accept ASCII, Base64 encoding is a common choice. JavaScript exposes btoa() to encode a “binary string” (each character’s code ⩽ 255) to Base64, and atob() for the reverse .
// Note: btoa/atob operate on “binary strings”
let binaryStr = "";
utf8bytes.forEach(byte => binaryStr += String.fromCharCode(byte));
// Encode to Base64
const base64 = btoa(binaryStr);
console.log(base64); // e.g., "SGVsbG8sIPCfkqA="
// Decode from Base64
const decodedBinary = atob(base64);
const decodedBytes = Uint8Array.from(decodedBinary, c => c.charCodeAt(0));
console.log(new TextDecoder().decode(decodedBytes)); // "Hello, 👋"
-
btoa() produces a Base64‑encoded ASCII string .
-
atob() decodes a Base64 string back to a binary string .
Bitwise Operations for Binary Manipulation
JavaScript’s bitwise operators let you inspect or modify individual bits of 32‑bit integers or BigInt values, treating them as sequences of ones and zeros . Common bitwise operations include:
-
& (AND), | (OR), ^ (XOR), ~ (NOT)
-
<< (left shift), >>
(sign‑propagating right shift), >>> (zero‑fill right shift)
let flags = 0b1101; // binary: 1101
flags &= 0b1011; // AND -> 1001 (9)
flags |= 0b0100; // OR -> 1101 (13)
flags ^= 0b0001; // XOR -> 1100 (12)
console.log(flags.toString(2)); // "1100"
-
The bitwise AND operator & returns bits set in both operands .
-
Shifts move bits left or right, inserting zeros or replicating the sign bit .
Putting It All Together: String → Binary → Base64 → String
Here’s a complete 4‑minute script demonstrating the flow:
<!DOCTYPE html>
<html>
<body>
<script>
// Original string
const original = "JS 🚀 Binary";
// 1. UTF-8 encoding
const encoder = new TextEncoder();
const utf8 = encoder.encode(original);
// 2. Build a “binary string”
let binStr = "";
utf8.forEach(b => binStr += String.fromCharCode(b));
// 3. Base64 encode
const b64 = btoa(binStr);
console.log("Base64:", b64);
// 4. Decode Base64
const decBin = atob(b64);
const decBytes = Uint8Array.from(decBin, c => c.charCodeAt(0));
// 5. UTF-8 decoding
const decoder = new TextDecoder();
const result = decoder.decode(decBytes);
console.log("Decoded:", result);
</script>
</body>
</html>
With just vanilla JavaScript, you’ve managed raw memory buffers, character‑encoding, Base64 conversion, and bitwise manipulation—all without external libraries!
Conclusion
This tutorial highlights the versatility of core JavaScript for performing tasks often associated with lower‑level languages. By mastering ArrayBuffer, TypedArrays, DataView, TextEncoder/TextDecoder, btoa/atob, and bitwise operators, you can confidently handle binary protocols, file formats, or any scenario requiring direct data manipulation in the browser or Node.js.
More Articles
Key Words:
JavascriptBonarybuffers developerArrayBuffer